Xamarin Setup
A complete guide to the basics of adding Nami to your Xamarin app.
This is a community supported project
The Nami Xamarin SDK is an incomplete and unofficial project created, but not maintained, by Nami ML.
We have provided a complete iOS binding library, a partially complete Android binding library, as well as an example app using Xamarin forms.
The project has known open issues tracked in GitHub that may prevent you from successfully integrating Nami into your Xamarin app.
Please feel free to contribute to the project by opening any new issues and creating pull requests with any proposed fixes!
Any accepted PRs will receive 6 months free of our Pro plan.
Please note that Nami support will not be able to help with Xamarin integrations.
Please make sure you have completed the setup of your app on the Nami Control Center for all these steps to be successful.
Adding Nami to your app has a few steps for a basic app.
- Add the SDK to your project
- Configure the SDK
- Show a paywall in your app
- React to a purchase to grant access to paid content or features
We'll run through each of these below.
Add the SDK to your project
Our Xamarin iOS binding library is available on GitHub and you can find the latest framework file that needs to be included at
https://packages.namiml.com/NamiSDK/Xamarin/<v.v.v>/Nami.framework.zip
by replacing <v.v.v>
with the correct version of the binding library.
The best way to add Nami to your project is to fetch our package from NuGet.
NuGet using Visual Studio for Mac
- Right-click on the Packages item under your Xamarin iOS Project and select Manage Packages.
- Type NamiML into the search box.
- Select the NamiML.SDK package from the list of NuGet packages.
- Click the Add Package button.
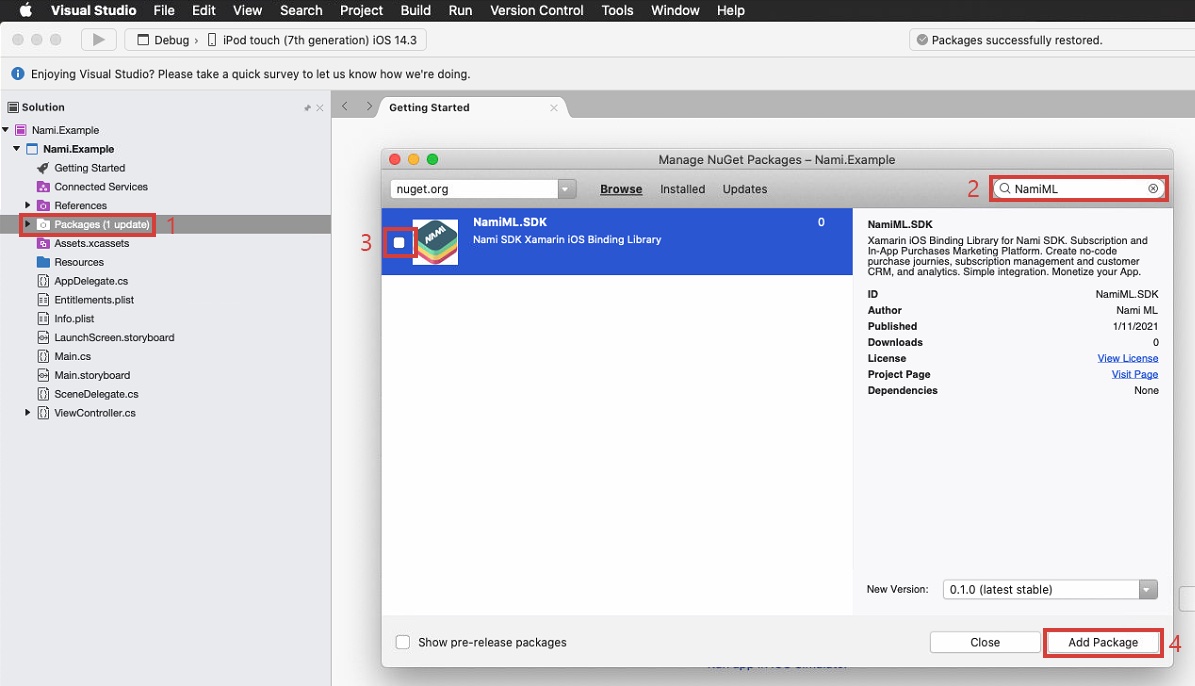
NuGet using Visual Studio on Windows
- Right-click on the References item under your Xamarin iOS Project and select Manage NuGet Packages....
- Type NamiML into the search box.
- Select the NamiML.SDK package from the list of NuGet packages.
- Click the Install button to add the package to your project.
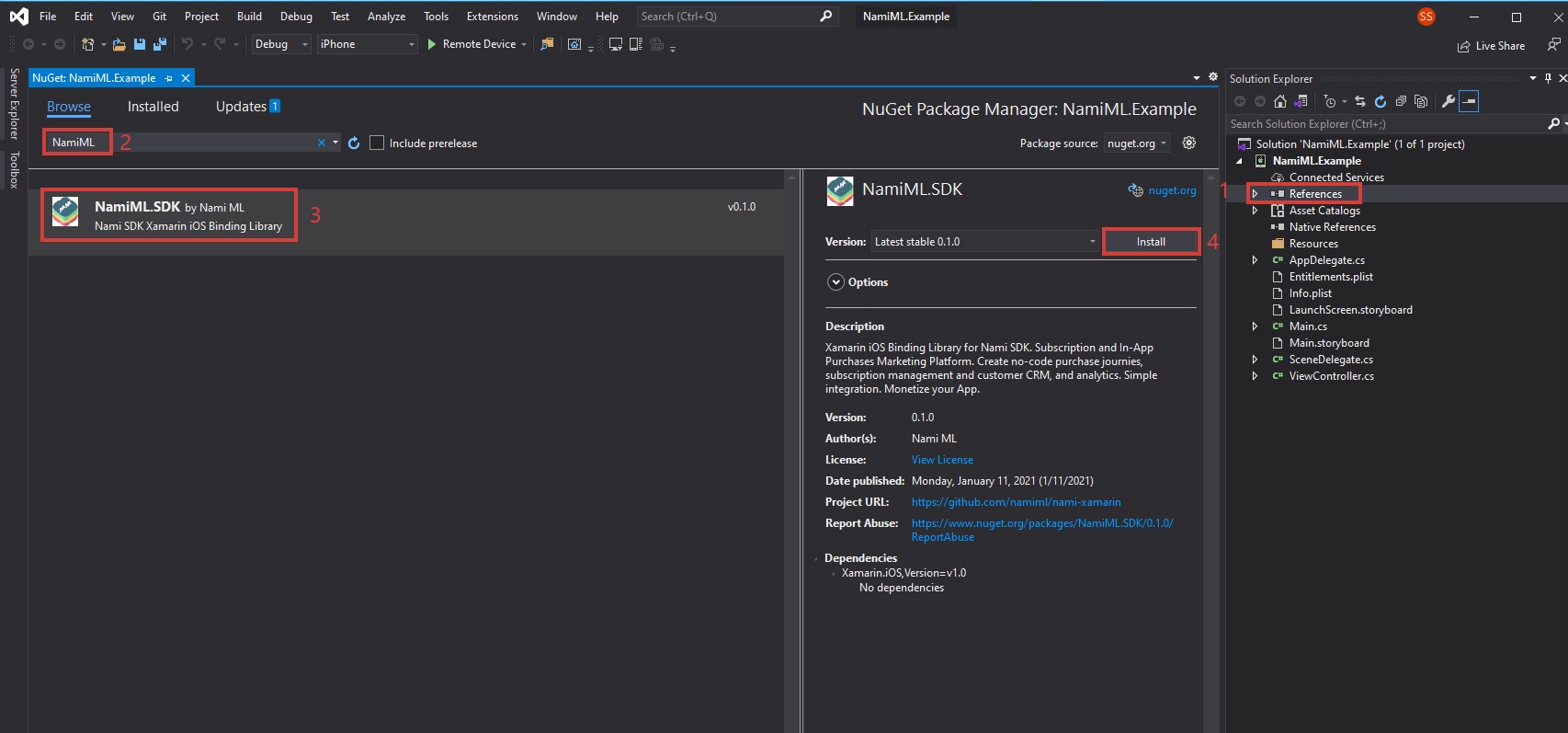
dotnet Command Line Interface
- Open terminal and navigate to the project directory.
- Type
dotnet add package NamiML.SDK
and hit enter.
Package Manager Console
- Select the Tools menu
- Click the NuGet Package Manager > sub-menu.
- Choose the Package Manager Console option from the sub-menu.
- Type in Install-Package NamiML.SDK and hit enter.
Configure the SDK
We recommend that you configure the Nami SDK as early in your app's launch as possible. This will ensure the SDK is ready to receive and process purchases.
The best spot to do this is in the FinishedLaunching
method in the AppDelegate.cs
file. We also recommend that you encapsulate the Nami configuration in its own method. Here's a full code example.
using NamiML;
[Register("AppDelegate")]
public class AppDelegate : UIResponder, IUIApplicationDelegate
{
[Export("application:didFinishLaunchingWithOptions:")]
public bool FinishedLaunching(UIApplication application, NSDictionary launchOptions)
{
NamiSetup();
Window = new UIWindow(UIScreen.MainScreen.Bounds);
var controller = new MainViewController();
Window.RootViewController = new UINavigationController(controller);
Window.MakeKeyAndVisible();
return true;
}
}
Now to setup Nami, let's look at the NamiSetup
method. You'll need to go find your App Platform ID in the Control Center for this step.
private void NamiSetup()
{
var namiConfig = NamiConfiguration.ConfigurationForAppPlatformID("YOUR_APP_PLATFORM_ID");
namiConfig.LogLevel = NamiLogLevel.Warn;
Nami.ConfigureWithNamiConfig(namiConfig);
}
Nami recommends setting the log level to Warn
for apps on the store. Info
may be helpful during development to better understand what is going on. Debug
level has a lot of information and is likely only helpful to the Nami support team.
Show a paywall
Now that you have the SDK configured, let's show a paywall in your app. This step requires that you have a Configured paywall in the Nami Control Center and that you have a live Campaign with a User-Initiated Paywall.
You may also optionally check if the SDK is able to raise the paywall at the time you are trying to display it.
if (NamiPaywallManager.CanRaisePaywall) {
NamiPaywallManager.RaisePaywall(this);
}
Grant access to paid app features
Once a user has made a purchase, you'll need to make sure to give them access to the content and features in your app that require a purchase. This is managed on the Nami platform through our entitlement engine.
The first option is to check whether a specific entitlement is active. This is done with the following code.
if (NamiEntitlementManager.isEntitlementActive("premium_access")) {
// allow access to premium app features
}
Nami also triggers a callback any time there is a change to the state of an entitlement. In the callback the full list of currently active entitlements is provided. You can use this to store the state of whether a user has access to premium features locally in your app.
It is important that any callbacks are created as early in the app launch as possible. We recommend adding the callback handlers in the NamiSetup
method in your AppDelegate.cs
.
NamiEntitlementManager.RegisterEntitlementsChangedHandler(changeHandler: (activeEntitlements) => {
foreach (var ent in activeEntitlements) {
var ent_id = ent.ReferenceID;
// use the active entitlements reference IDs to grant
// access to premium app features
}
});
That's all the basics to get up and running with Nami using Xamarin. For more use cases, explore the rest of our docs.
Updated about 2 years ago